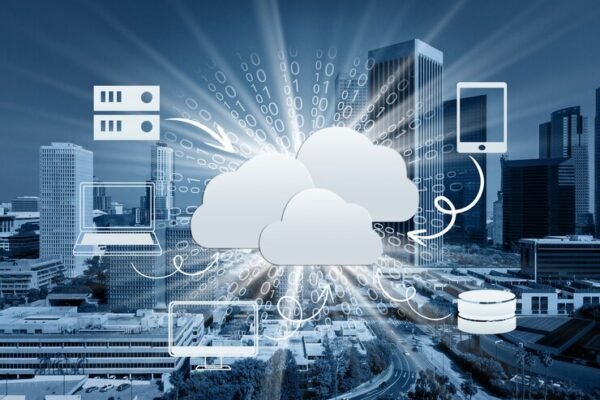
Why Your Business Needs Microsoft Azure Cloud Service in UAE Today
As the UAE continues to position itself as a global hub for innovation, technology, and business, the need for companies to adopt advanced digital solutions has never been greater. Businesses are increasingly looking for ways to modernize their infrastructure, optimize operations, and scale their services efficiently. Microsoft Azure Cloud Service in UAE offers a comprehensive…